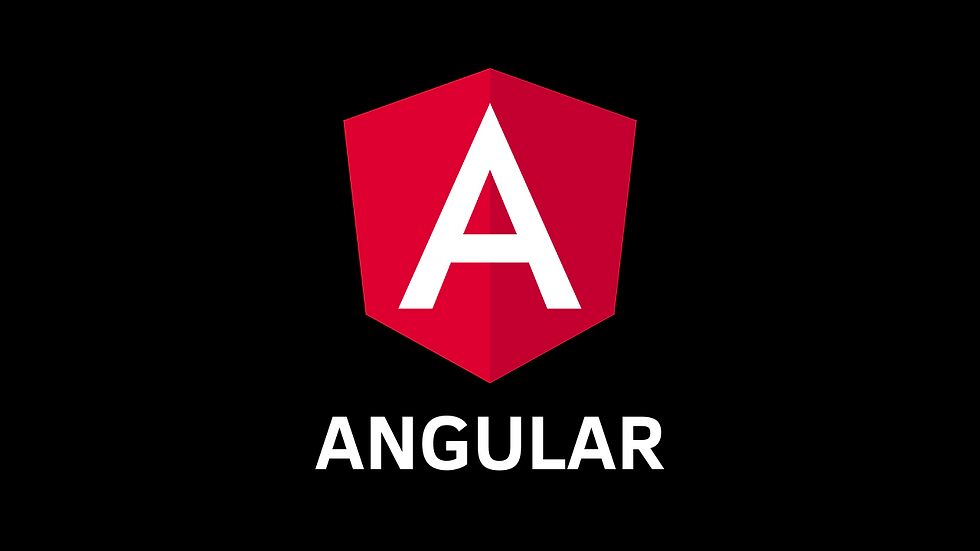
Introduction
Recently the latest trend has been reactive forms in Angular, so I decided to write this blog with a detailed step-by-step explanation of a simple input form with validations.
Prerequisites:
Installation of Angular 8 CLI, refer to my blog here
Step-by-Step explanation:
Step 1: Command to create the new project in angular
ng new angular-reactive-form
Step 2: Add ReactiveFormsModule references to app.module.ts as below
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { ReactiveFormsModule } from '@angular/forms';
import { AppRoutingModule } from './app-routing.module';
import { AppComponent } from './app.component';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
ReactiveFormsModule,
AppRoutingModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
Step 3: Add below code to build the form with validations in app.component.ts
import { Component, OnInit } from '@angular/core';
import { FormGroup, FormControl, Validators } from '@angular/forms';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit{
signupForm: FormGroup;
ngOnInit(){
this.signupForm=new FormGroup({
userdata:new FormGroup({
'username': new FormControl(null,Validators.required),
'email': new FormControl(null, [Validators.required, Validators.email]),
'password' : new FormControl(null,Validators.required)
})
})
}
onSubmit(){
console.log(this.signupForm);
}
onReset(){
this.signupForm.reset();
}
}
Step 4: Now lets build the form structure with below code in app.component.html
<div class="container">
<div class="row">
<div class="col-xs-12 col-sm-10 col-md-8 col-sm-offset-1 col-md-offset-2">
<form [formGroup]="signupForm" (ngSubmit)="onSubmit()">
<div formGroupName="userdata">
<div class="form-group">
<label for="username">Username</label>
<input
type="text"
id="username"
formControlName="username"
class="form-control">
<span
*ngIf="!signupForm.get('userdata.username').valid && signupForm.get('userdata.username').touched"
class="help-block"
>Please enter the username!</span>
</div>
<div class="form-group">
<label for="email">Email</label>
<input
type="text"
id="email"
formControlName="email"
class="form-control">
<span
*ngIf="!signupForm.get('userdata.email').valid && signupForm.get('userdata.email').touched"
class="help-block"
>Please enter the email!</span>
</div>
</div>
<div class="form-group">
<label for="password">Password</label>
<input
type="password"
id="password"
formControlName="password"
class="form-control">
<span
*ngIf="!signupForm.get('userdata.password').valid && signupForm.get('userdata.password').touched"
class="help-block"
>Please enter the password!</span>
</div>
<span
*ngIf="!signupForm.valid && signupForm.touched"
class="help-block"
>Form is invalid!</span>
<button class="btn btn-primary" type="submit">Submit</button>
<button class="btn btn-primary buttonStyle" (click)="onReset()" type="button">Reset</button>
</form>
</div>
</div>
</div>
Step 4: A wee bit of styling with below code in app.component.css
.container {
margin-top: 30px;
background-color: azure;
}
.buttonStyle{
margin-left: 10px;
}
Step 5: Serve the code with ng serve command, below is a short demonstration
Hope you found this useful.
Sharing is Caring!
Thanks for reading!
Comments